What is Room Database in Android
INTRODUCTION
- Room is a constant library that is part of the Android jetpack. It is built On the top of SQLite. The room persistent library has many advantages over raw SQLite.
- Room makes everything easy and clear to create a Database and achieve the operations on it.
- Database to save and perform the operations on endless data locally. The Room DataBase is suggested by Google over SQLite.
- It can help to manage data.
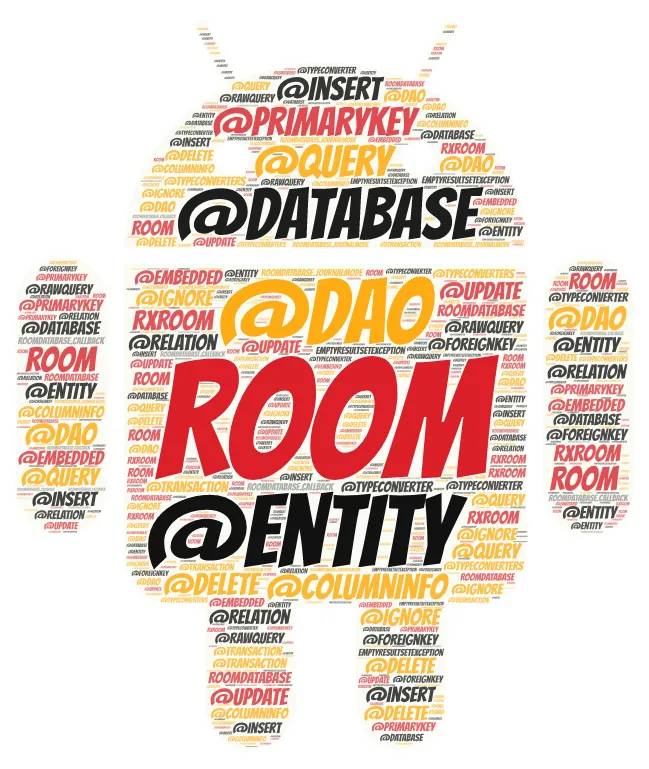
WHY USE ROOM?
- Cache the applicable pieces of the data so that when the user’s device is offline, they can still browse and aspect the content offline.
- Compile-time SQLite query verification protects the application from collision.
- The comment that it gives minimises the boilerplate code.
CONDITIONS IN ROOM DATABASE-
- Have Android Studio installed.
- Have a basic knowledge of building Android applications.
- Have a basic understanding of the Kotlin programming language, SQL, MVVM architecture and Kotlin coroutines.
KEY POINTS OF ROOM DATABASE–
- No need to write rough Queries.
- Compile Time verification of SQL queries.
- This quickly supports the merging with other Architecture components.
- Room provides an easier way to work with LiveData and perform the operations.
- There is no need to change the code when the database schema gets changed.
PRIMARY COMPONENTS OF ROOM–
- Database Class: This provides the main access point to the basic connection for the application’s persisted data. This is annotated with @Database.
- Data Entities: This represents all the tables in the existing Database. This is annotated with @Entity.
- DAO: It stands for DATA ACCESS OBJECT. This contains the method to perform the operations on the Database. This is annotated with @DAO.
- @Insert-It is Used to insert records into the Room Database.
- @Delete-It is Used to delete records into the Room Database.
- @Update-It is Used to update records in the Room Database.
- @Query-It is Used to enter the Query like(SELECT FROM).
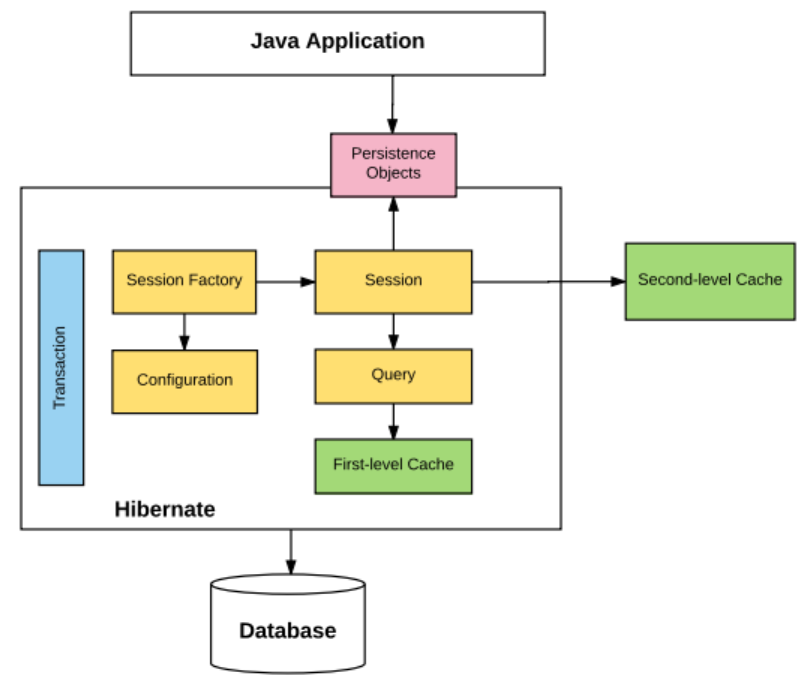
Steps to implement Room Database in Android Application-
Step 1: Create an Empty Activity project
- Firstly We can Create an empty Android Studio project. Create/Start a New Project in Android Studio.
Step 2: Adding the Required Dependencies
- Then We Can Add the dependencies to the app-level gradle file. By going to ProjectName -> src -> build. gradle.
Step 3: Creating Data Entity
- Create a Sample Data class named User. kt.
- And call on the code which contains entities User as an entity, which represents a row and first_name, last_name, and age represent column names of the table.
Step 4: Creating Data Access Objects (DAOs):
- Now create an interface named UserDao.kt.
- And call on the code which provides various methods which are used by the application to interact with the user.
Step 5: Creating the Database
- Now creating the Database which defines the real application’s database, which is the main access point to the application’s continuous data. This class must satisfy:
- The class must be abstract.
- The class should be annotated with @Database.
- Database class must define an abstract method with zero arguments and return a case of DAO.
- Now call on the code inside the AppDatabase.kt file.
Step 6: Usage of the Room Database
- Inside the MainActivity.kt file we can create a database, by providing usage names for the database.
Leave a Reply